Python 3.10 introduced several new features aimed at enhancing productivity and code readability. Among these, the match
statement stands out as one of the most significant additions, offering a powerful tool for structural pattern matching. This feature, often likened to a combination of if
, elif
, and else
statements, provides a more expressive way to handle conditional logic based on the structure of data types.
Understanding Structural Pattern Matching
At its core, structural pattern matching allows developers to inspect and manipulate complex data structures in a declarative manner. It enables the program to automatically extract values from data structures that match specified patterns, significantly reducing boilerplate code and improving code readability.
Key Concepts of the Match Statement
- Literal Patterns: These are straightforward comparisons where the value of an expression is checked against a specific literal value.
- Variable Patterns: Allows extracting values from data structures and binding them to variables.
- Class Patterns: Enables matching instances of specific classes.
- Guard Clauses: Conditions that must be satisfied for a pattern to match.
- Nested Patterns: Combining multiple patterns to match nested data structures.
Practical Examples of Using the Match Statement
Let’s delve into some practical examples to illustrate how the match
statement can be utilized in various scenarios:
Matching Literal Values
value = "apple"
match value:
case "banana":
print("Banana is healthy.")
case "apple":
print("Apple is tasty.")
case _:
print("Unknown fruit.")
Extracting Values from Tuples
point = (1, 2)
match point:
case (x, y):
print(f"Point is at ({x}, {y}).")
case _:
print("Invalid point format.")
Handling Nested Structures
person = {"name": "Alice", "age": 30}
match person:
case {"name": name, "age": age}:
print(f"Hello, {name}. You are {age} years old.")
case _:
print("Invalid person format.")
Advantages of Using the Match Statement
- Readability: The
match
statement makes code easier to understand by clearly expressing intent through patterns. - Simplicity: Reduces the complexity of handling multiple conditions and data structure manipulations.
- Flexibility: Supports advanced pattern matching techniques, including guards and nested patterns, providing a powerful tool for data manipulation.
Conclusion
The introduction of the match
statement in Python 3.10 represents a significant leap forward in the language’s ability to handle complex data structures and conditional logic. By leveraging structural pattern matching, we can write more concise, readable, and maintainable code.
Whether you’re dealing with simple literals or complex nested data structures, the match
statement offers a versatile and expressive way to manage conditional logic in Python.
As Python continues to evolve, features like the match
statement underscore the language’s commitment to innovation and developer empowerment.
Bonus! Example
import sys
while True:
cmd = input("Enter command ")
match cmd.split():
case ["quit"]:
break
case ["hello"]:
print("Hello World")
case ["help"]:
print("Commands include [quit, help, hello]")
case _:
print("Unknown command")
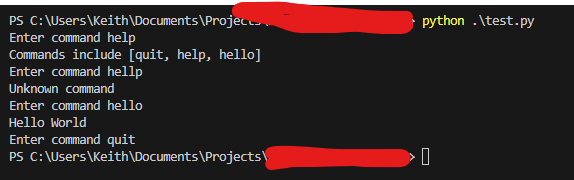