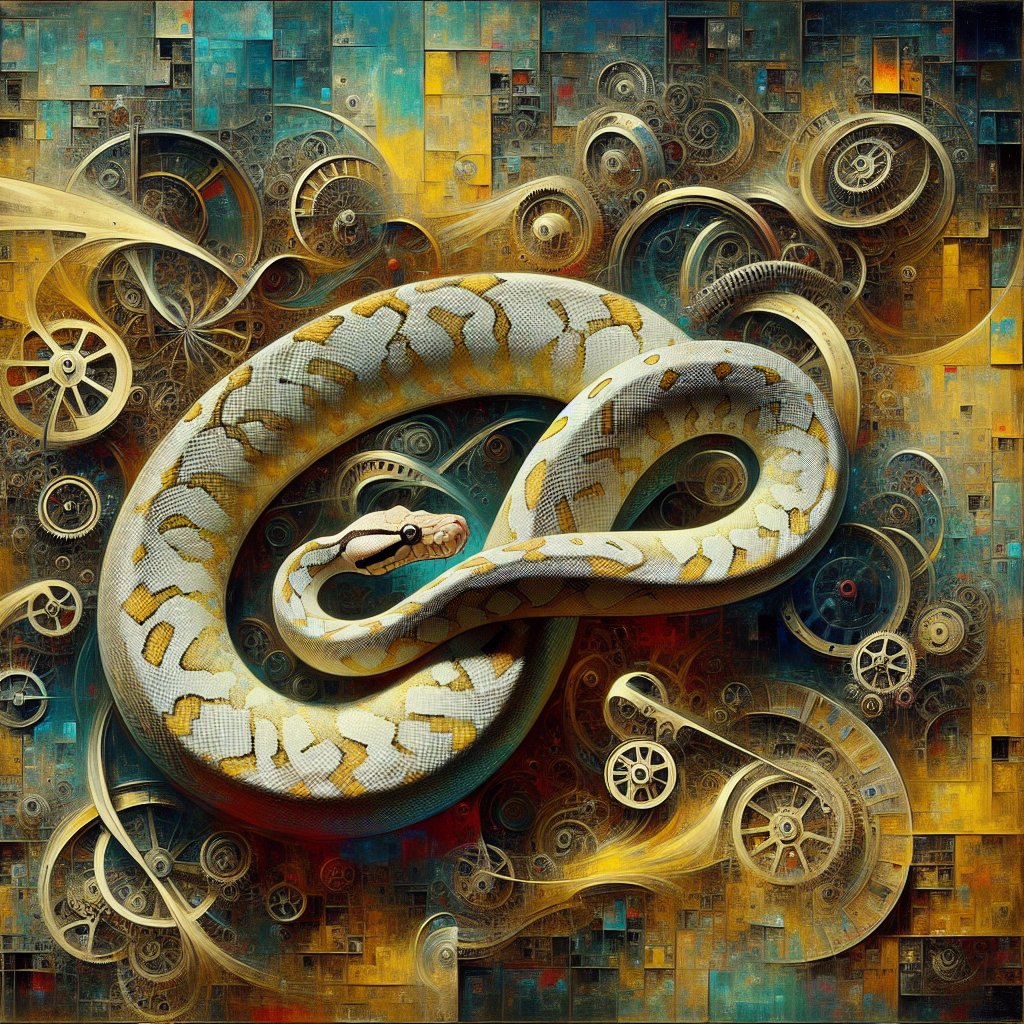
Recently, i was exploring the use case of having python trigger a dynamic ‘python’ payload on the fly with no knowledge of what the payload might contain, other than the required func name in it called handler().
This was my first attempt at building this concept. Note, this is an extremely bad thing to do in production and is highly sensitive to path traversal attacks as well as numerous other “holes”. Do Not Do This for production applications.
The Code, is relatively simple and is exposed below. This basically expects a UTF-8 encoded string param containing the payload to be passed when the function is called. As an example, a simple payload could look like this..
string_py = """
def handler():
return 'Hello World'
"""
resp = trigger_py(string_py)
The main logic below would be called, and execute the dynamic payload above – capturing the output and returning it back in the function response.
import re
import subprocess
def trigger_py(body):
script_str = body.decode("utf-8")
script_globals = {}
# Regular expression to match import statements
import_regex = re.compile(r'^import (.+)$')
lines = script_str.split('\n')
for line in lines:
match = import_regex.match(line) # Check if the line is an import statement
if match:
module_name = match.group(1).strip() # Extract the module name from the import statement
try: # Attempt to dynamically import the module
exec(f"import {module_name}", script_globals)
except (ImportError, ModuleNotFoundError):
# If the import fails, try to install the module
try:
subprocess.check_call(['python', '-m', 'pip', 'install', '--user', module_name])
# After installation, retry importing the module
exec(f"import {module_name}", script_globals)
except (subprocess.CalledProcessError, ModuleNotFoundError) as e:
print(f"Failed to install {module_name}: {e}")
return f"Error: Failed to install required package {module_name}"
else:
exec(line, script_globals)
exec(script_str, script_globals)
result = script_globals['handler']()